TypeScript is a powerful language that adds static typing to JavaScript, making it a safer and more reliable choice for building applications. However, this added safety can sometimes lead to error messages that might seem confusing, especially for newcomers. One such error is ‘TS2532: Object is possibly undefined.’
This tutorial teaches you the reasons and the steps to solve the Error TS2532: Object is possibly undefined in TypeScript.
Table of Contents
Understanding the Error TS2532: Object is possibly undefined
The ‘TS2532: Object is possibly undefined’ error occurs when TypeScript identifies a potential issue with null or undefined values. TypeScript is designed to help catch runtime errors during development, and this error message alerts you to a possible problem in your code.
Here’s an example of the error in action:
const obj: { name: string } | undefined = undefined;
console.log(obj.name);
This codedeclares a variable called obj
of type { name: string } | undefined
.
This means that obj
can either be an object with a name
property of type string
, or it can be undefined.
The code then sets obj
to undefined. This means that obj
is now undefined, and it does not have a name
property.
Finally, the code tries to log the name
property of obj
to the console. However, since obj
is undefined, it does not have a name
property, and this line of code will throw the error TS2532: Object is possibly undefined
.
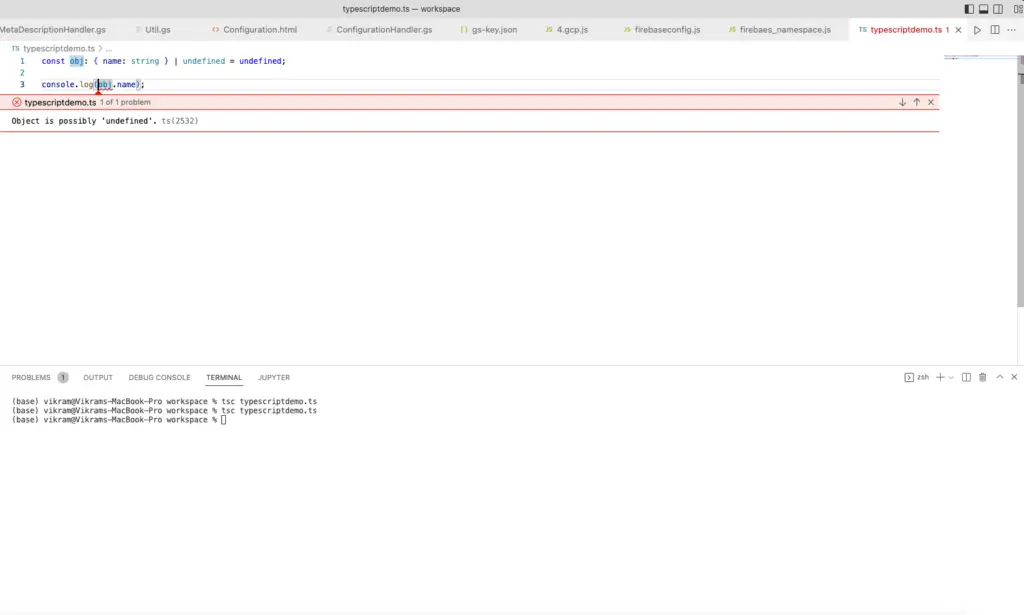
Resolving the Error TS2532: Object is possibly undefined
Now that we understand what the error tells us, let’s explore how to resolve it.
Declare and Initialize the object
This is the most straightforward way to ensure that the object is defined.
const obj: { name: string } = { name: "John Doe" };
Use Optional Chaining (TypeScript 3.7+)
If you are using TypeScript 3.7 or newer, you can use optional chaining to safely access properties on possibly undefined objects. This prevents the error and provides a default value if the object is undefined.
const name = newUser?.name || 'Guest'; // Use optional chaining and provide a default
console.log(`Hello, ${name}`);
Type Assertions
If you are certain that an object exists, but TypeScript is unable to infer it, you can use a type assertion to tell TypeScript explicitly about the object’s type.
const newUser = { age: 25 } as { name: string };
greet(newUser); // No error because of the type assertion
Check for Null/Undefined
You can also use conditional checks to ensure that an object is not null
or undefined
before accessing its properties.
if (newUser) {
greet(newUser);
} else {
console.log('User is missing.');
}
Use Non-null Assertion Operator (!)
In cases where you are sure that an object is not null
or undefined
, you can use the non-null assertion operator (!
) to tell TypeScript not to raise an error.
const newUser!: { name: string }; // Use '!' to assert non-null
greet(newUser); // No error is raised
Use a Guard clause
A guard clause is a conditional statement that is used to check whether the object is defined before accessing it.
const obj: { name: string } | undefined;
if (obj) {
console.log(obj.name); // This will only log the name property if the object is defined.
}
Check Object Properties:
The error often occurs when you try to access properties on an object without ensuring that the object exists. To fix this, ensure the object has the expected properties or handle cases where the object might be undefined
.
function greet(user: { name: string }) {
console.log(`Hello, ${user.name}`);
}
const newUser = { name: 'Alice' }; // Provide a 'name' property
greet(newUser);
Conclusion
The ‘TS2532: Object is possibly undefined’ error in TypeScript is helpful for catching potential issues with null or undefined values in your code. By understanding the error message and applying the suggested solutions, you can write safer and more reliable TypeScript code. Remember always to handle cases where objects might be undefined and use TypeScript’s features to your advantage to prevent runtime errors in your applications.